RabbitMQ is a powerful message broker widely used for managing asynchronous communication between services. While it provides a reliable messaging system, transient failures and processing errors can still occur. To ensure message reliability and prevent data loss, implementing retries and dead letter queues (DLQs) is essential.
In this blog, we’ll explore how to use RabbitMQ effectively to handle errors, retries, and dead letter queues.
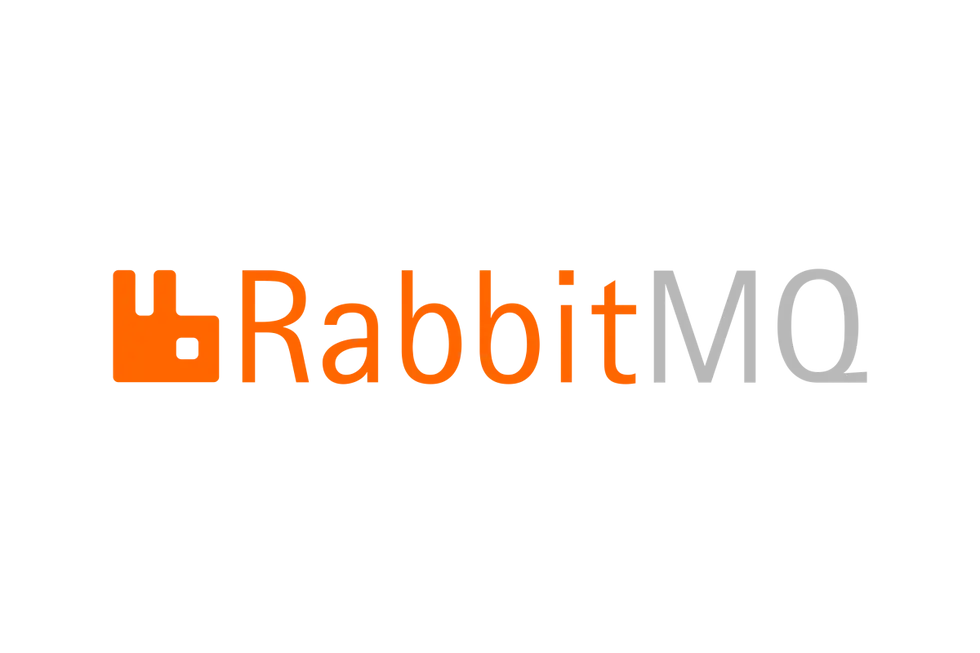
Why Use RabbitMQ for Reliable Messaging?
RabbitMQ ensures message delivery between producers and consumers, but failures—such as network issues, crashes, or unhandled exceptions—can cause messages to fail processing. By using retries and DLQs, we can:
Avoid message loss by ensuring failed messages are retried.
Prevent infinite retry loops by directing repeatedly failing messages to a DLQ.
Improve observability by tracking and investigating problematic messages.
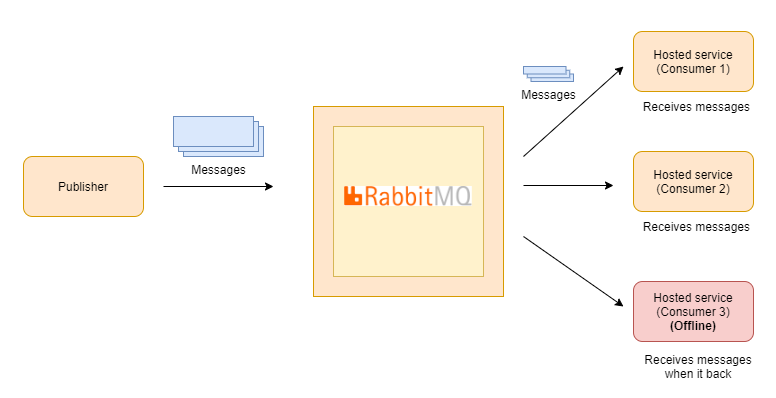
Setting Up a Retry Mechanism in RabbitMQ
1. Creating the Main Queue
We first define the main queue where messages are initially published.
$channel->queue_declare('main_queue', false, true, false, false, false, [
'x-dead-letter-exchange' => ['S', 'retry_exchange']
]);
Messages that fail processing in main_queue will be routed to retry_exchange.
2. Configuring a Retry Queue
A retry queue introduces a delay before retrying message processing.
$channel->queue_declare('retry_queue', false, true, false, false, false, [
'x-dead-letter-exchange' => ['S', 'main_exchange'],
'x-message-ttl' => 60000 // Delay of 60 seconds before retrying
]);
Here, x-message-ttl defines how long a message stays in the queue before being sent back to main_exchange for reprocessing.
3. Implementing a Dead Letter Queue (DLQ)
When a message repeatedly fails, we move it to a DLQ for further investigation.
$channel->queue_declare('dead_letter_queue', false, true, false, false);
$channel->exchange_declare('dead_letter_exchange', 'direct', false, true, false);
$channel->queue_bind('dead_letter_queue', 'dead_letter_exchange');
4. Publishing Messages with Retry Handling
Whenever an error occurs during message processing, the message is re-queued instead of being lost.
$callback = function ($msg) use ($channel) {
try {
// Process message
echo "Processing: " . $msg->body . "\n";
throw new Exception("Simulated processing error"); // Simulating failure
} catch (Exception $e) {
echo "Error processing message: " . $msg->body . " - Retrying\n";
$channel->basic_publish($msg, 'retry_exchange');
}
};
$channel->basic_consume('main_queue', '', false, true, false, false, $callback);
while ($channel->is_consuming()) {
$channel->wait();
}
5. Handling Dead Letter Messages
To process failed messages in the DLQ, set up a consumer for monitoring and alerting.
$callbackDLQ = function ($msg) {
echo "Dead Letter Queue Message: " . $msg->body . "\n";
file_put_contents('/var/logs/dead_letter.log', $msg->body . "\n", FILE_APPEND);
};
$channel->basic_consume('dead_letter_queue', '', false, true, false, false, $callbackDLQ);
while ($channel->is_consuming()) {
$channel->wait();
}
Key Benefits of This Approach
Increased Fault Tolerance – Ensures messages are retried instead of being dropped.
Better Debugging – DLQ enables tracking of permanently failing messages.
Scalability – Efficiently handles message processing in high-throughput environments.
Conclusion
RabbitMQ, when configured with retries and dead letter queues, provides a robust system for handling failures in message processing. By using these techniques, you can build resilient, scalable, and fault-tolerant applications.
Have you used RabbitMQ’s retry and DLQ mechanisms in your projects? Share your experiences in the comments below!
コメント